Tutorial on sending commands and receiving answers
In this tutorial we are going to learn how to use the Blue Seal Basic Controller to send commands to a Python script running on any platform and catch responses via Wi-Fi network.

The main idea of this tutorial is to create a program running on a computer which waits for text commands arriving at some port (i.e., $(COMPORT)) via TCP/IP protocol. The commands will be in form of a string and can obtain various information. The program can read the text meassages, process them and with respect to their content carry out different tasks. Such a program will be from its nature called server.
The program used to send the commands will be client and will connect to the server in order to send the command and then disconnect. Our client is the Blue Seal (Basic) Controller running on an Android device.
In the next three chapters we will learn how to start the server using a simple Python script, operate the Blue Seal Controller and how to use the BS Controller to send commands to the server and display received data from the server.
The Python Script
The most basic script for Python running a TCP/IP server will be the following
#!/usr/bin/python ###------------------------------------------------------------------ ### This code is a part of a tutorial for the Blue Seal Project ### created by Jiří Daněk (www.bluesealproject.com). ### Please, do not remove this remark from the code. ###------------------------------------------------------------------ # First, import necessary libraries import sys, SocketServer # We have to set the IP address of the computer and the port used HOST, PORT = "192.168.0.21", 9999 # Now, we define class and define the TCP/IP server within the class class MyTCPHandler(SocketServer.BaseRequestHandler): """ The RequestHandler class for our server. It is instantiated once per connection to the server, and must override the handle() method to implement communication to the client. """ def handle(self): # self.request is the TCP socket connected to the client self.data = self.request.recv(1024).strip() # write out the IP address of the connected client print("{} wrote:".format(self.client_address[0])) # write out the message sent by the connected client decoded with UTF-8 print(self.data.decode("utf-8")) """ Next command sends back received data, but the lower-cased, in the format the BS Basic Controller can process and display """ self.request.sendall("$(RTN=" + self.data.lower() + ")") # Create the server, binding to localhost on port 9999 server = SocketServer.TCPServer((HOST, PORT), MyTCPHandler) # Start the main program if __name__ == "__main__": # Activate the server; this will keep running until you # interrupt the program with Ctrl-C server.serve_forever()
The code can be dowloaded here.
The script creates a TCP/IP server at IP address 192.168.0.21 (i.e., IP address of the computer) and port 9999 waiting for a client. When the client connects and sends a message, the scripts writes out the IP address of the client and the received message (e.g., "DIAL_01"). Further, the server converts the message into lower-case letters and sends it bact to the client in the form (i.e., "$(RTN=dial_01)").
The Blue Seal Controller
First of all, we have to reset the Blue Seal Controller to the Basic settings (i.e., go to settings and set Settings/General/Predefined Settings -> Basic Settings). One has to be careful since restoring the Basic Settings discards all changes made by the user!
As the next step, one has to connect the Android device running the BS Controller to the same network as the TCP/IP server and set the values as shown in previous section Tutorials for the BS Controller.
Using the BS Controller along with the Python script
In this part we are going to describe in a little more detail how does the communication between the BS Basic Controller and the TCP/IP server created with Python.
Preparing to run the tutorial
Here comes a short list of task which has to be done before proceding to the communicational part. Please, respect the order of the tasks.
Tasks to do first:- Starting network and connecting to it
- create a Wi-Fi network
- connect a PC with installed Python to the Wi-Fi network
- connect an Android device with installed Blue Seal Controller to the network
- Setting and starting the TCP/IP server on PC
- in the Python script (given above) change the value of HOST to match the IP address of the PC in the network (we have shown in the prevous section how to obtain the address)
- save the change and run the script in Python
- Setting and starting the Blue Seal Controller on Android phone
- start the Blue Seal Controller on your device
- make sure you have the Basic Settings (as described in this section above)
- go to Settings/General and set the IP address of the robot (i.e., value for $(IP_R)) so it matches the value of HOST from the Python script (i.e., the IP address of the PC)
- if necesary, change the value of the communication port (i.e., $(COMPORT)) in Settings/General to match the value PORT in the Python script
- start the Blue Seal Basic Controller
Running the tutorial: communication between the PC and the Android phone
If you followed and executed all the tasks writen above, you should see on your Android device the very same screen as on the left of the following picture (i.e., 1.).
Congratulations! Now you are ready to command the PC with your Android phone!
Let us now describe all the steps, so we understand the way the BS Controller and the Python script operate.
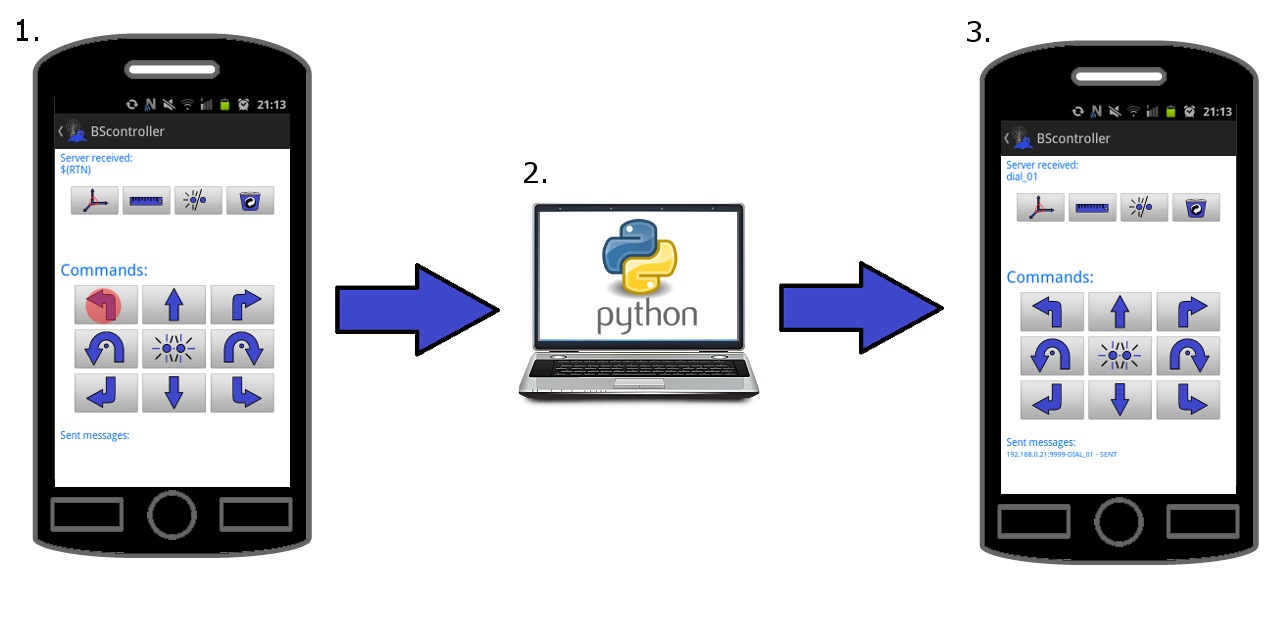
Let us now describe how the communication works:
-
- Right after the start of the app, the text field on the top of the screen contains still a key $(RTN). The key will be later substituted by appropriate value from the server's response.
- Pressing the button DIAL_01 (denoted by a red dot on the picture) sends a command to the server on the IP address $(IP_R) on the port $(COMPORT).
- The sent command is of the form "$(CMD)" where the key $(CMD) is substituted by the string defined in Settings/BS Basic Controller/Dial-Up Buttons/Dial-Up Button 01 -> Set Command ($(CMD)).
-
- The TCP/IP server on PC receives the command from the app BS Basic Controller.
- The script prints on the PC's screen the IP address of the client (i.e., the Android device running the BS Controller)
- The script prints the received string on the PC's screen.
- The script transforms the received string into lower-case and sends it back as string "$(STR=received_text_lower_case)".
-
- The BS Controller receives answer from the server and writes out in the text field on the bottom the sent command with detailed informations.
- The BS Controller uses all keys defined in the mask for received (defined in Settings/BS Basic Controller/Set Mask) to search for keys' values which can appear in the answer from the server. In this case the key $(STR) will be substituted by the value received_text_lower_case from the server's answer defined above.
- Note!
In case of any troubles with the network, an error message will be displayed in the text field on the bottom of the screen.
Perfect! Now we know how to command your computer with an Android phone!
Before we conclude, let us make some final remarks on various features (if you find them too confusing, you can skip them).
Remarks:
- Now, the Python script opens doors to uncountable possibilities for extensions.
- The value $(CMD) can be set for any button individually. It can even contain other keys (e.g., $(STEPS), $(STR_A) or $(IP_C) ).
Before the individual commands are sent, they are substituted in to a mask (defined in Settings/BS Basic Controller/Set Mask). In this way, a more general and uniform form of the commands can be defined.
All the keys are replaced by the appropriate values before the command is sent to the server. - The mask for the received commands can contain multiple keys which will be then substituted by the appropriate values from the server's answer or an empty string if the values are not in the answer. This feature can be used to receive various informations from the PC.
Conclusion
In this section we have learnt how to create a TCP/IP server on a PC using Python. Further, we have learned how to use the Blue Seal Controller to connect to the server as a client in order to send commands and receive answers from the server which are then displayed on the screen of the BS Basic Controller.
Although, the tutorial provided here is fairly simple, it provides many possibilities for extensions satisfying various user's demands.
Share this page with friends via